
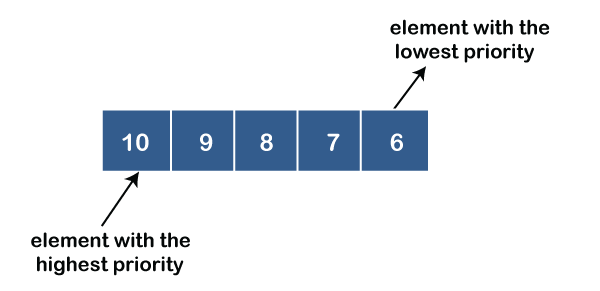
For example, if the graph costs are ints and the heuristic returns a double, then you need the priority queue to accept doubles. edges: dict] = ĭef cost( self, from_node: GridLocation, to_node: GridLocation) -> float: The type of the priority values including the type used in the priority queue should be big enough to include both the graph costs (costt) and the heuristic value. What does a graph look like? It’s a location type along with a class with a method to get neighboring locations: On this page, I’ll fill in the rest of the details to make complete working programs. In the main article, I focused on search. Queue a data structure used by the search algorithm to decide the order in which to process the graph locations. Search an algorithm that takes a graph, a starting graph location, and optionally a goal graph location, and calculates some useful information (reached, parent pointer, distance) for some or all graph locations. They may include additional information such as direction, fuel, lane, or inventory, depending on the problem being solved. As you can see, the size of the queue is 3 now because we have removed one element from it. getting elements from queue Queue.get () get size of priority queue in python print ( 'the size of queue is:', Queue.qsize ()) Output: bash. These are not necessarily locations on the map. The get method returns the element from the queue. Locations a simple value (int, string, tuple, etc.) that labels locations in the graph. A weighted graph also gives a cost of moving along an edge. These are the abstractions I’ll use: Graph a data structure that can tell me the neighbors for each graph location (see this tutorial).
#PRIORITY QUEUE PYTHON EXAMPLE CODE#
The main article shows the Python code for the search algorithm, but we also need to define the graph it works on. Let’s implement Breadth First Search in Python. These use Python 3 so if you use Python 2, you will need to remove type annotations, change the super() call, and change the print function to work with Python 2. There are a few extra bits that you can find in implementation.py. Treat the code on this page as a starting point, not as a final version of the algorithm that works for all situations.
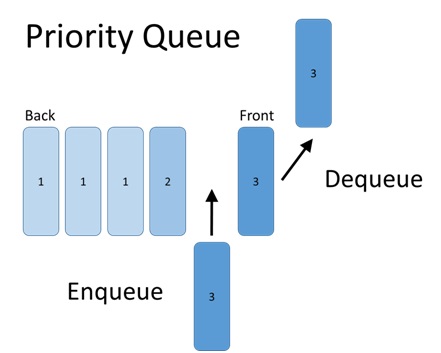
There are lots of variants of the algorithms, and lots of variants in implementation. Graph search is a family of related algorithms.
#PRIORITY QUEUE PYTHON EXAMPLE HOW TO#
On this page I show how to implement Breadth-First Search, Dijkstra’s Algorithm, Greedy Best-First Search, and A*. heapq works on the principle of binary heap, while. heapq heapifies the original list inplace, while using PriorityQueue it doesn’t modify the original data. PriorityQueue implements locking to ensure thread safety, thus it is slower than heapq. This article is a companion guide to my introduction to A*, where I explain how the algorithms work. Python queue PriorityQueue is thread-safe, but heapq doesn’t guarantee thread safety.
